How do I perform client-side browser-based uploads with Wasabi
- 22 Aug 2024
- 1 Minute to read
- Print
- PDF
How do I perform client-side browser-based uploads with Wasabi
- Updated on 22 Aug 2024
- 1 Minute to read
- Print
- PDF
Article summary
Did you find this summary helpful?
Thank you for your feedback
There are several ways one can implement a web page upload directly into Wasabi using scripts. Companies can use this method to allow their employees perform uploads and submit their work through the company designed client-side webpage and not allow employees perform direct server-side uploads.
The below example demonstrates the same using html javascript code for uploading files to Wasabi from a web page.
Note that this code example discusses the use of Wasabi's us-east-1 storage region. To use other Wasabi storage regions, please use the appropriate Wasabi service URL as described here.
<!DOCTYPE html>
<html>
<head>
<script src="https://sdk.amazonaws.com/js/aws-sdk-2.283.1.min.js"></script>
</head>
<body>
<h1>Wasabi Upload Test</h1>
<input type="file" id="wasabiupload" onchange="handleFile()" />
</body>
<script>
function handleFile() {
// console.log("handle file - " + JSON.stringify(event, null, 2));
var files = document.getElementById('wasabiupload').files;
if (!files.length) {
returnalert('Please choose a file to upload first.');
}
var f = files[0];
var fileName = f.name;
const s3 = new AWS.S3({
correctClockSkew:true,
endpoint:'https://s3.us-east-1.wasabisys.com', //Specify the correct endpoint based on where your bucket is
accessKeyId:'Wasabi-Access-Key',
secretAccessKey:'Wasabi-Secret-Key',
region:'us-east-1'//Specify the correct region name based on where your bucket is
,logger: console
});
console.log('Loaded');
const uploadRequest = new AWS.S3.ManagedUpload({
params: { Bucket:'Wasabi-Bucket-Name', Key:fileName, Body:f },
service:s3
});
uploadRequest.on('httpUploadProgress', function(event) {
const progressPercentage = Math.floor(event.loaded * 100 / event.total);
console.log('Upload progress ' + progressPercentage);
});
console.log('Configed and sending');
uploadRequest.send(function(err) {
if (err) {
console.log('UPLOAD ERROR: ' + JSON.stringify(err, null, 2));
} else {
console.log('Good upload');
}
});
}
</script>
</html>
Here's how the output looks for the above script:
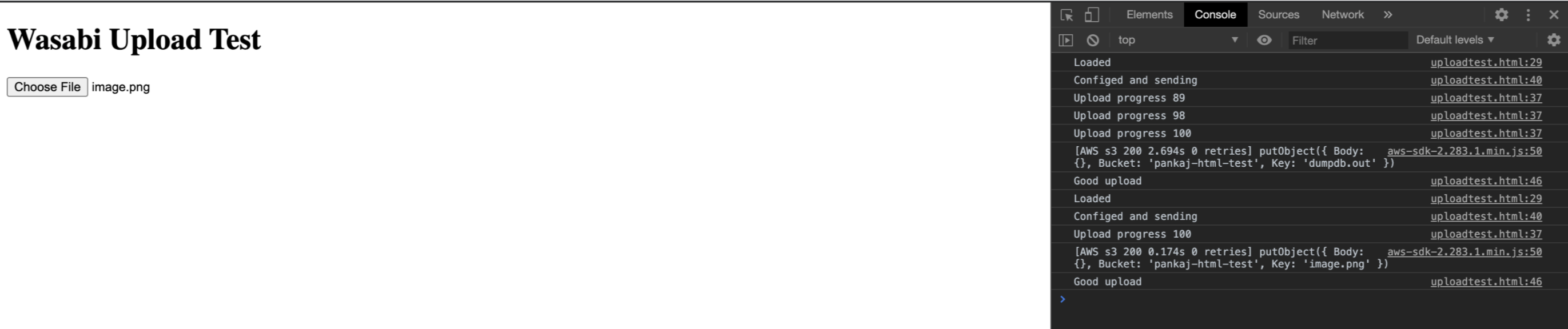